Salesforce Developer Interview Questions – Apex Triggers Continued
APEX – TRIGGERS CONTINUED…
Table of Contents
72. Can we call batch apex in trigger?
Answer:
Yes, we can call batch apex in trigger.
73. Can a trigger make a call to the apex callout method?
Answer:
We can call a callout method in apex trigger but the only condition is that it has to be an asynchronous callout because the trigger flow cannot wait on the response received by the callout method.
We should use a future method to call the callout method in triggers.
74. Can we call a scheduled class from the trigger?
Answer:
Yes we can but it is not recommended.
75. What is a recursive Trigger?
Answer:
A recursive trigger is the one which calls itself over and over again and eventually exceeds the maximum trigger depth limit.
76. How to avoid the recursive trigger?
Answer:
To avoid recursive triggers we can create a class with a static Boolean variable and default it to true. In the trigger, before executing the code check if the static variable is true or not . Once executed, make the variable false.
So, when the trigger tries to execute next time the static variable will be false and hence it will not execute the trigger again.
But be mindful before implementing a recursive trigger as sometimes we might need a trigger to execute more than one.
77. How to show the error messages on the page or on the field using Trigger. (similar to validation rules error) ?
Answer:
We can use addError method in triggers to show the message on the page or on the field
Example
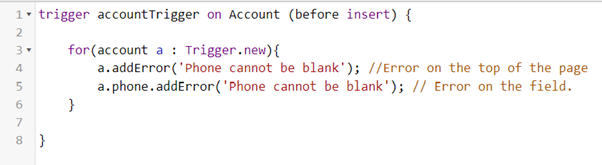
78. When an account is created then automatically 3 contacts should be created .On what object we should write the trigger ?
Answer:
Trigger should be written on Account
79. When an account is created then automatically 3 contacts should be created. What type of trigger should it be? (Before or After)
Answer:
After insert.
80. How many triggers can we create on an object?
Answer:
We can create as many triggers as we want on an object but it is recommended to create one trigger per object.
81. What are bulk triggers ? or How to bulkify triggers?
Answer:
A trigger handling multiple records at a time is a bulk trigger.
All triggers are bulk triggers by default, and can process multiple records at a time. We should always write triggers which can handle more than one record at a time.
82. What are trigger handlers?
Answer:
Trigger handler is an apex class to handle the logic of a trigger. It is used to provide a better way of writing complex logic that’s required for trigger code and also to avoid creating more than one trigger per object.
When we write the trigger handler class then the execution or trigger point starts in the trigger code but the actual logic is written in handler class.
Use the Trigger handler to write all the logic or code and create your triggers as logic-less triggers.
Trigger Code
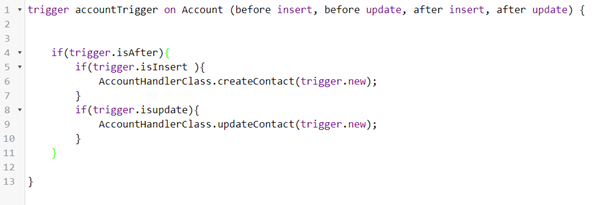
Trigger Handler Class Code
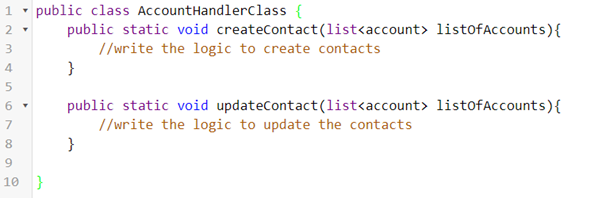
83. What are helper classes?
Answer:
A helper class is also a class which is used to write common methods which can be used by multiple other classes.
Example:
- To find the weather of a city
- Calculate the tax for a salary/income
- To find the country of a city
84. What are triggers best practices?
Answer:
1) One Trigger Per Object
A single Apex Trigger is all you need for one particular object. If you develop multiple Triggers for a single object, you have no way of controlling the order of execution if those Triggers can run in the same contexts
2) Logic-less Triggers
If you write methods in your Triggers, those can’t be exposed for test purposes. You also can’t expose logic to be re-used anywhere else in your org.
3) Context-Specific Handler Methods
Create context-specific handler methods in Trigger handlers
4) Bulkify your Code
Bulkifying Apex code refers to the concept of making sure the code properly handles more than one record at a time.
5) Avoid SOQL Queries or DML statements inside FOR Loops
An individual Apex request gets a maximum of 100 SOQL queries before exceeding that governor limit. So if this trigger is invoked by a batch of more than 100 Account records, the governor limit will throw a runtime exception
6) Using Collections, Streamlining Queries, and Efficient For Loops
It is important to use Apex Collections to efficiently query data and store the data in memory. A combination of using collections and streamlining SOQL queries can substantially help writing efficient Apex code and avoid governor limits
7) Querying Large Data Sets
The total number of records that can be returned by SOQL queries in a request is 50,000. If returning a large set of queries causes you to exceed your heap limit, then a SOQL query for loop must be used instead. It can process multiple batches of records through the use of internal calls to query and queryMore
8) Use @future Appropriately
It is critical to write your Apex code to efficiently handle bulk or many records at a time. This is also true for asynchronous Apex methods (those annotated with the @future keyword). The differences between synchronous and asynchronous Apex can be found
9) Avoid Hardcoding IDs
When deploying Apex code between sandbox and production environments, or installing Force.com AppExchange packages, it is essential to avoid hardcoding IDs in the Apex code. By doing so, if the record IDs change between environments, the logic can dynamically identify the proper data to operate against and not fail
85. Explain Triggers order of execution?
Answer:
- Loads the original record from the database or initializes the record for an upsert statement.
- Loads the new record field values from the request and overwrites the old values.
If the request came from a standard UI edit page, Salesforce runs system validation to check the record for:- Compliance with layout-specific rules
- Required values at the layout level and field-definition level
- Valid field formats
- Maximum field length
- When the request comes from other sources, such as an Apex application or a SOAP API call, Salesforce validates only the foreign keys. Before executing a trigger, Salesforce verifies that any custom foreign keys don’t refer to the object itself.
Salesforce runs custom validation rules if multiline items were created, such as quote line items and opportunity line items. - Executes record-triggered flows that are configured to run before the record is saved.
- Executes all before triggers.
- Runs most system validation steps again, such as verifying that all required fields have a non-null value, and runs any custom validation rules. The only system validation that Salesforce doesn’t run a second time (when the request comes from a standard UI edit page) is the enforcement of layout-specific rules.
- Executes duplicate rules. If the duplicate rule identifies the record as a duplicate and uses the block action, the record isn’t saved and no further steps, such as after triggers and workflow rules, are taken.
- Saves the record to the database, but doesn’t commit yet.
- Executes all after triggers.
- Executes assignment rules.
- Executes auto-response rules.
- Executes workflow rules. If there are workflow field updates:
- Updates the record again.
- Runs system validations again. Custom validation rules, flows, duplicate rules, processes, and escalation rules aren’t run again.
- Executes before update triggers and after update triggers, regardless of the record operation (insert or update), one more time (and only one more time)
- Executes escalation rules.
- Executes the following Salesforce Flow automations, but not in a guaranteed order.
- Processes
- Flows launched by processes
- Flows launched by workflow rules (flow trigger workflow actions pilot)
- When a process or flow executes a DML operation, the affected record goes through the save procedure.
- Executes record-triggered flows that are configured to run after the record is saved.
- Executes entitlement rules.
- If the record contains a roll-up summary field or is part of a cross-object workflow, performs calculations and updates the roll-up summary field in the parent record. Parent record goes through save procedure.
- If the parent record is updated, and a grandparent record contains a roll-up summary field or is part of a cross-object workflow, performs calculations and updates the roll-up summary field in the grandparent record. Grandparent record goes through save procedure.
- Executes Criteria Based Sharing evaluation.
- Commits all DML operations to the database.
- After the changes are committed to the database, executes post-commit logic are executed. Examples of post-commit logic (in no particular order) include:
- Sending email
- Enqueued asynchronous Apex jobs, including queueable jobs and future methods
- Asynchronous paths in record-triggered flows